Sunday, August 27, 2006
Giving Back...
I hope that I can contribute back to this community by submitting a few bugs and posting a few ideas, thoughts or discoveries that I come up with while working and having fun.
Friday, August 25, 2006
Sun Updates Java Tutorials Website

The update seems to have a lot to do with upcoming release of JDK6, because the content already reflects the new features included in this release. Either way, it is nice to have one place to go to get some more insight into Java core libraries and tools and the proper way of using them. Some parts of the tutorials I've checked out were a little bit too "high-level", but that is understandable considering the scope of JDK. The only critisizm I have about the site is that it could use some "face lifting", but that might change by the time JDK6 is released.
Sunday, August 20, 2006
Debugging Deadlocks with JConsole in JDK6

If a code is deadlock prone, it doesn't mean that the deadlock will occur. Deadlock will occur only when wrong actions take place at a wrong time (or order), which makes it really difficult to find and debug deadlocks. When an application is deadlocked it appears to do nothing while consuming only very little of CPU time. The chance of a deadlock appearing grows with the number of threads that execute deadlock prone code as well as with the number of repetitive executions of deadlock prone code (even if it is being executed by only a few threads). The conclusion of this is that if you stress your code by multi-threaded tests, it is more likely that a deadlock will appear compared to no testing, or testing the code only with single-threaded test cases. If a deadlock appears, the tests will freeze and that's about everything you will see. To track down what caused the deadlock is usually very difficult at this point.
JConsole comes to rescue
JConsole - Java Monitoring & Management Console, is a utility that is a part of the JDK since version 5.0 and has been greatly enhanced in upcoming JDK 6. JConsole is JMX based which enables it to easily connect to running JVM and monitor application running in JVM. The only requirement is to start JVM with parameter
com.sun.management.jmxremote
as:
java -Dcom.sun.management.jmxremote YourLocalApp
or
java -Dcom.sun.management.jmxremote.port=portNum YourRemoteApp
Once the JVM is running you can launch JConsole and connect to the JVM and start monitoring.
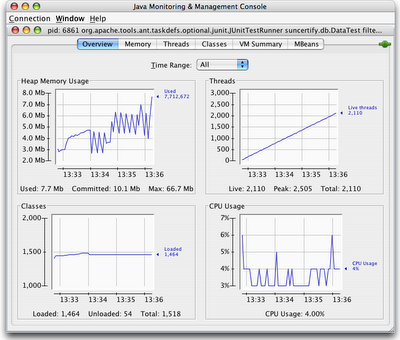
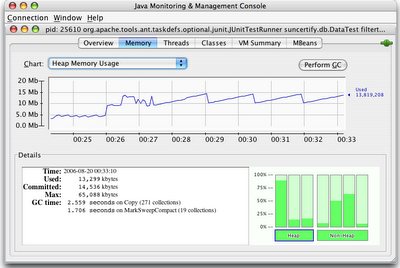
One of the features that has been enhanced is "Deadlock detection" located in "Threads" tab. Simply described: if your application gets deadlocked, you can start up the JConsole and see where the deadlock occurred, which threads are involved, and which thread owns and is blocked for which locks. Pretty cool :)

Wednesday, August 16, 2006
Java Singleton Revised

In this article the author describes problems with different Java implementations of singleton pattern that are most of the time visible only in multi-threaded environments.
To make long story short his conclusion is that it is not good practice writing a singleton class as:
class Singleton { private static Singleton instance; private Singleton() { //initialize instance } public static Singleton getInstance() { if (instance == null) instance = new Singleton(); return instance; } }
This is commonly used but as described in the article, not a thread safe implementation. Developers should neither use a double-checked locking implementation (described in the article), but rather use one of the two options:
- make
getInstance()
method synchronized - which will be thread safe, but will have impact on performance - initialize static class variable
instance
at declaration time like this:
class Singleton { private static Singleton instance = new Singleton(); private Singleton() { //initialize instance } public Singleton getInstance() { return instance; } }
I like simple solutions to problems that are not always simple and so I like this solution. However IMHO, this implementation is not suitable for all cases where you might want to use singleton pattern.
Let's take a singleton that executes operations on a file as an example. At some point in your program you need to close the RandomAccessFile
, FileOutputStream
or FileWriter
that you are using (you always close files and db connections right? :) ), so I would expect that you would add another static method to the Singleton
class that would close all necessary resources that you opened in the private constructor.
Let's call this method closeInstance()
and implement it like this:
public static synchronized void closeInstance() { //close all necessary resources instance = null; }
We closed all resources and since these resources (required for this class to operate) are closed, we can also set the instance to null
to prevent someone using Singleton
instance while it is an in unusable state. This method is synchronized to prevent some problems with concurrent access.
What will happen if you try to call getInstance()
now? A null
will be returned. That is not really what you would expect, is it? What would you do if for whatever reason you closed the Singleton
instance and later on realized that you need to open it again. You don't have access to constructor and no other way how to initialize the singleton again.
My solution is based on the solution of Peter Haggar, but enhances it to prevent the issues I mentioned above.
public class Singleton { private static Singleton instance = new Singleton(); private Singleton() { //initialize instance //opens all necessary resources } /** * Returns the singleton instance. If the singleton instance was * not previously closed, the instance is initialized before first * call of this method automatically. * * @throws IllegalStateException if instance had been closed by *closeInstance()
method */ public static Singleton getInstance() { if (instance == null) throw new IllegalStateException( "Singleton instance has been closed already"); return instance; } /** * Cleanly closes singleton resources and removes the singleton * instance */ public static synchronized void closeInstance() { //close all necessary resources instance = null; } /** * Creates and returns a reference to the new instance. * If the instance already exists, then the reference to this * instance is returned. This method is intended to be used only * in cases when there is a chance that the instance was previously * closed. In other cases use ofgetInstance()
is encouraged because * of performance benefits, since this method is synchronized * as opposed togetInstance()
. */ public static synchronized Singleton createInstance() { if (instance == null) instance = new Singleton(); return instance; } /** * Checks the status of the singleton instance * * @return true if singleton instance is initialized, if instance * was closed returns false. */ public static boolean instanceCreated() { return instance != null; } }
This implementation of Singleton gives you all performance benefits of the first mentioned implementation while being thread safe and giving you all the flexibility of reinitializing the singleton instance once it was previously, intentionally destroyed.